以下のようなプロジェクトを作成して下さい。
項目 | 設定内容 |
---|---|
Project Name | greeting |
Applecation Name | greeting |
Package Name | jp.co.example.greet |
アクティビティの作成 | MainActivity |
ビルドターゲット | Android 2.2 |
ワーキングセットにプロジェクトを追加 | チェックする |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#aaaaaa" > <RadioGroup android:id="@+id/time" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical"> <RadioButton android:id="@+id/morning" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#000000" android:text="朝" /> <RadioButton android:id="@+id/afternoon" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#000000" android:text="昼" /> <RadioButton android:id="@+id/night" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#000000" android:text="晩" /> </RadioGroup> <Button android:id="@+id/great" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="挨拶をする" /> <TextView android:id="@+id/result" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#000000" android:textColor="#FFFFFF" android:textSize="20sp" android:gravity="center" /> </LinearLayout>
public class MainActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //--------------------------------------------------------------------------------------------- // ↓ここから //--------------------------------------------------------------------------------------------- final RadioGroup time = (RadioGroup) findViewById(R.id.time); Button great = (Button) findViewById(R.id.great); final TextView result = (TextView) findViewById(R.id.result); great.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String message = ""; switch (time.getCheckedRadioButtonId()) { case R.id.morning: message = "おはよう"; break; case R.id.afternoon: message = "こんにちは"; break; case R.id.night: message = "こんばんは"; break; } result.setText(message); } }); //--------------------------------------------------------------------------------------------- //↑ ここまで //--------------------------------------------------------------------------------------------- } }
このように表示されましたか? 「朝」「昼」「晩」のいづれかを選択し、挨拶をするボタンを押してみて下さい。
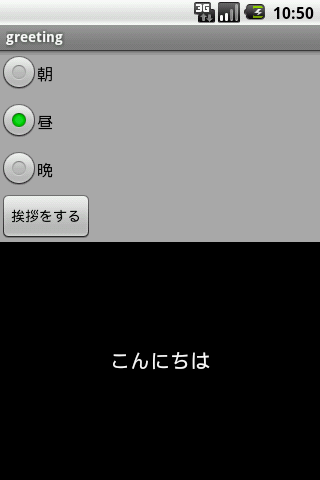
以下のようなプロジェクトを作成して下さい。
項目 | 設定内容 |
---|---|
Project Name | calculation |
Applecation Name | 足し算 |
Package Name | jp.co.example.calc |
アクティビティの作成 | CalculationActivity |
ビルドターゲット | Android 2.2 |
ワーキングセットにプロジェクトを追加 | チェックする |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" android:paddingTop="10dp" android:background="#a0a0a0" > <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:background="#a0a0a0"> <EditText android:id="@+id/value1" android:layout_width="100dp" android:singleLine="true" android:numeric="signed" android:layout_height="wrap_content" /> <TextView android:layout_width="30dp" android:layout_height="wrap_content" android:gravity="center" android:textColor="#000000" android:text="+" /> <EditText android:id="@+id/value2" android:layout_width="100dp" android:singleLine="true" android:numeric="signed" android:layout_height="wrap_content" /> </LinearLayout> <Button android:id="@+id/calc" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textColor="#000000" android:text="計算する" /> </LinearLayout>
public class CalculationActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //--------------------------------------------------------------------------------------------- // ↓ここから //--------------------------------------------------------------------------------------------- final EditText value1 = (EditText) findViewById(R.id.value1); final EditText value2 = (EditText) findViewById(R.id.value2); Button btn = (Button) findViewById(R.id.calc); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // 計算するボタン押下時に、キーボードを消す InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE); inputMethodManager.hideSoftInputFromWindow(v.getWindowToken(), 0); SpannableStringBuilder v1 = (SpannableStringBuilder) value1 .getText(); String val1 = v1.toString(); SpannableStringBuilder v2 = (SpannableStringBuilder) value2 .getText(); String val2 = v2.toString(); Long ans; try { ans = Long.parseLong(val1) + Long.parseLong(val2); } catch (NumberFormatException e) { // トーストを表示 Toast.makeText(CalculationActivity.this, e.toString(), Toast.LENGTH_SHORT).show(); return; } // LogCatに出力 Log.i("anster", String.valueOf(ans)); // トーストを表示 Toast.makeText(CalculationActivity.this, "計算結果は " + String.valueOf(ans) + " です", Toast.LENGTH_SHORT).show(); } }); //--------------------------------------------------------------------------------------------- //↑ ここまで //--------------------------------------------------------------------------------------------- } }
このように表示されましたか? 実際に、値を入力し、「計算する」ボタンを押してみてください。
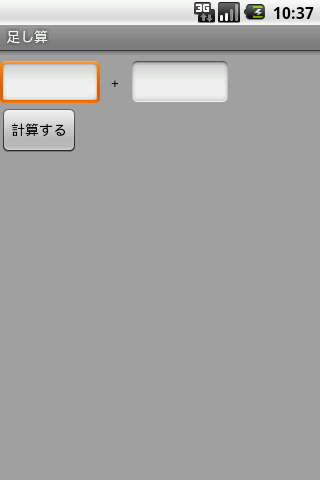
このページへのコメント
rogans dhgate terrariums
http://download.imtoo.zune.video.converter.3.1.sof...
px7QMF <a href="http://paheqcqshyvs.com/">paheqcqshyvs</a>, [url=http://aqpsnhiscrzv.com/]aqpsnhiscrzv[/url], [link=http://lapneuisnfpj.com/]lapneuisnfpj[/link], http://bisostztxewi.com/
http://pfrfdcbmaqow.com/
WvwbFi <a href="http://fwfqozuaudxp.com/">fwfqozuaudxp</a>, [url=http://frwydwssghco.com/]frwydwssghco[/url], [link=http://wdywoyjgtapx.com/]wdywoyjgtapx[/link], http://doqnixmvifst.com/
http://ncuaetkfauvy.com/
uqS0I7 <a href="http://srmvxewyagcd.com/">srmvxewyagcd</a>, [url=http://kgrmtktxfudq.com/]kgrmtktxfudq[/url], [link=http://ooyaaedboebg.com/]ooyaaedboebg[/link], http://tycaszljnqoi.com/