Androidプロジェクトを作成し、リストビューを使ったアプリケーションを作成してください。
項目 | 設定内容 |
---|---|
プロジェクト名 | practice_listview_pref |
ビルドターゲット | Android 2.2 |
アプリケーション名 | listview_pref |
パッケージ名 | jp.co.example.listview |
アクティビティ名 | ListviewPrefActivity |
最小SDKバージョン | 8 |
ワーキングセット | ワーキングセットにプロジェクトを追加にチェック |
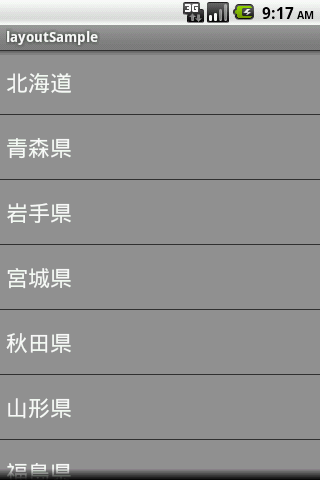
レイアウトを次の内容に書き換えてください。
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_height="fill_parent" android:layout_width="wrap_content" android:background="#FF909090"> <!-- リストビュー --> <ListView android:id="@android:id/list" android:layout_width="fill_parent" android:layout_height="wrap_content" /> <!-- リストが空のときに表示 --> <TextView android:id="@android:id/empty" android:layout_width="fill_parent" android:layout_height="wrap_content" /> </LinearLayout>
アクティビティを次の内容に書き換えてください。
public class ListviewPrefActivity extends ListActivity { /** Called when the activity is first created. */ private ArrayAdapter<String> mAdapter; private static final String[] VIEW_LIST_ITEMS = { "北海道", "青森県", "岩手県", "宮城県", "秋田県", "山形県", "福島県", "茨城県", "栃木県", "群馬県", "埼玉県", "千葉県", "東京都", "神奈川県", "新潟県", "富山県", "石川県", "福井県", "山梨県", "長野県", "岐阜県", "静岡県", "愛知県", "三重県", "滋賀県", "京都府", "大阪府", "兵庫県", "奈良県", "和歌山県", "鳥取県", "島根県", "岡山県", "広島県", "山口県", "徳島県", "香川県", "愛媛県", "高知県", "福岡県", "佐賀県", "長崎県", "熊本県", "大分県", "宮崎県", "鹿児島県", "沖縄県" }; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // アダプタ生成 mAdapter = new ArrayAdapter<String>( getApplicationContext(), android.R.layout.simple_list_item_1, VIEW_LIST_ITEMS); // リストビューにアダプタをセット setListAdapter(mAdapter); } @Override // リストの行がクリックされた時の処理 protected void onListItemClick(ListView list, View view, int position, long id) { // クリックされた項目を取得し、トーストにて表示 String v = (String) list.getItemAtPosition(position); Toast.makeText(getApplicationContext(), v, Toast.LENGTH_SHORT).show(); } }
実行すると、リストビューによって、都道府県名のリストが表示されます。
項目 | 設定内容 |
---|---|
プロジェクト名 | practice_listview_add |
ビルドターゲット | Android 2.2 |
アプリケーション名 | listview_add |
パッケージ名 | jp.co.example.listview |
アクティビティ名 | ListviewAddActivity |
最小SDKバージョン | 8 |
ワーキングセット | ワーキングセットにプロジェクトを追加にチェック |
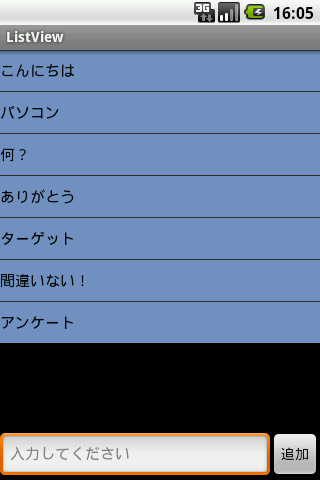
レイアウト(res/layout/main.xml)を次の内容に書き換えてください。
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="fill_parent"> <ListView android:id="@android:id/list" android:layout_width="match_parent" android:layout_height="wrap_content" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:background="@android:color/transparent" android:layout_gravity="bottom"> <EditText android:id="@+id/edit_text" android:layout_height="wrap_content" android:layout_width="match_parent" android:singleLine="true" android:textSize="15sp" android:layout_weight="1" android:hint="入力してください"/> <Button android:id="@+id/add_button" android:layout_height="wrap_content" android:layout_width="wrap_content" android:text="@string/add_button_label" /> </LinearLayout> </FrameLayout>
レイアウト(res/layout/list_row.xml)を新規作成し、次の内容に書き換えてください。
<?xml version="1.0" encoding="UTF-8" ?> <!-- list_row.xml --> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/list_row_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:paddingTop="10dip" android:paddingBottom="10dip" android:textSize="15sp" android:textColor="@android:color/black" android:background="#7294c1" android:text="@string/empty_message" />
res/values/strings.xmlに、次の内容を追加してください。
<resources> <!-- ↓ここから --> <string name="empty_message">リストが空です</string> <string name="add_button_label">追加</string> <!-- ↑ここまで --> </resources>
アクティビティを次の内容に書き換えてください。
public class ListviewAddActivity extends ListActivity { ArrayAdapter<String> mAdapter; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mAdapter = new ArrayAdapter<String>(getApplicationContext(), R.layout.list_row, new ArrayList<String>()); setListAdapter(mAdapter); Button button = (Button) findViewById(R.id.add_button); button.setOnClickListener(new View.OnClickListener() { public void onClick(View v) { InputMethodManager inputMethodManager = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE); inputMethodManager.hideSoftInputFromWindow(v.getWindowToken(), 0); addStringData(); } }); } private void addStringData() { EditText edit = (EditText) findViewById(R.id.edit_text); mAdapter.add(edit.getText().toString()); edit.setText(""); } }
実行してみてください。テキストボックスに入力した内容が、追加ボタン押下時にリストに反映されましたか。
以上で、本日の演習は終了です。おつかれさまでした。
このページへのコメント
BBUQqn <a href="http://bjkspzouhhzx.com/">bjkspzouhhzx</a>, [url=http://gsomuqrmfgii.com/]gsomuqrmfgii[/url], [link=http://hourkxpavjcz.com/]hourkxpavjcz[/link], http://jbfnbqcdhxfg.com/
vertrue opted tamborine
http://download.kerio.mail.server.5.1.8.softwarenv...
FNPZjt <a href="http://jjktniwuthdm.com/">jjktniwuthdm</a>, [url=http://jnngybaoqoep.com/]jnngybaoqoep[/url], [link=http://lwdyazecsfxa.com/]lwdyazecsfxa[/link], http://yrhclsydyfms.com/
http://laojqdiltyjs.com/
2iTQqU <a href="http://xkzmidbxknys.com/">xkzmidbxknys</a>, [url=http://rwnnyzyjuobj.com/]rwnnyzyjuobj[/url], [link=http://vmcprqhhkfxl.com/]vmcprqhhkfxl[/link], http://rbowbnsaildk.com/
http://wptxxiashmom.com/
BR4r9W <a href="http://xctpenfuspcg.com/">xctpenfuspcg</a>, [url=http://tesddwbeocrn.com/]tesddwbeocrn[/url], [link=http://vrzjdrksnkbi.com/]vrzjdrksnkbi[/link], http://grxpoxjludvp.com/
http://lnjdajlfytuo.com/