画面上に、ビュー(テキストビュー、ラジオボタンなど)を配置するために使われます。 ひとつのビューグループの中には、複数のビューまたはビューグループを配置でき 全体として階層構造(ツリー構造)をとることができます。 これによって、複雑なレイアウトを作成することが可能になっています。
名称 | クラス | 概要 |
---|---|---|
リニアレイアウト | LinearLayout | 水平方向または垂直方向にビューを配置する。 |
相対レイアウト | RelativeLayout | このビュー同士の相対関係や、親のビューの相対関係で配置する。 |
フレームレイアウト | FrameLayout | 左上を基点に、ひとつのビューを配置する。複数のビューを重ね合わせることも可能。 |
テーブルレイアウト | TableLayout | テーブル形式にビューを配置する。通常、内部にテーブル行レイアウトを指定する。 |
属性値 | 意味 |
---|---|
top | 上寄せにする |
bottom | 下寄せにする |
left | 左寄せにする |
right | 右寄せにする |
center_vertical | 垂直方向の中央に配置する |
center_horizontal | 水平方向の中央に配置する |
fill_vertical | 垂直方向をコンテナのサイズに拡大して配置する |
fill_horizontal | 水平方向をコンテナのサイズに拡大して配置する |
center | 水平方向と垂直方向の中央に配置する |
fill | 水平方向と垂直方向をコンテナのサイズに拡大して配置する |
※right|bottomのように、2つの指定を「|」でつなぐ事も可能
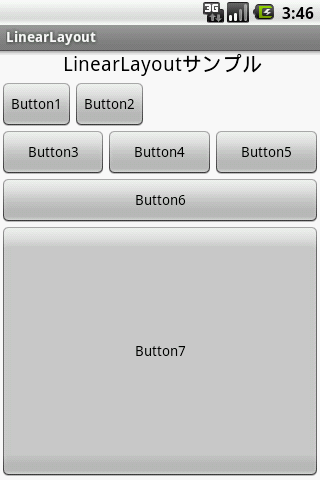
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/white"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:textSize="20dip" android:paddingBottom="5dip" android:textColor="@android:color/black" android:text=" LinearLayoutサンプル" /> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button1" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button2" /> </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button3" android:layout_weight="1" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button4" android:layout_weight="1" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Button5" android:layout_weight="1" /> </LinearLayout> <Button android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Button6" /> <Button android:layout_width="fill_parent" android:layout_height="fill_parent" android:text="Button7" /> </LinearLayout>
属性 | 意味 |
---|---|
layout_above | 指定したビューの上に配置する |
layout_below | 指定したビューの下に配置する |
layout_toLeftOf | 指定したビューの左に配置する |
layout_toRightOf | 指定したビューの右に配置する |
layout_alignBaseline | 指定したビューのベースラインに合わせて配置する |
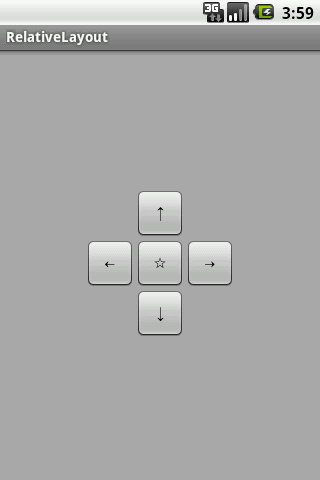
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/darker_gray" android:padding="20dip"> <Button android:id="@+id/center" android:layout_width="50dip" android:layout_height="50dip" android:layout_centerInParent="true" android:text="☆" /> <Button android:id="@+id/avobe" android:layout_width="50dip" android:layout_height="50dip" android:layout_above="@+id/center" android:layout_alignLeft="@+id/center" android:text="↑" /> <Button android:id="@+id/below" android:layout_width="50dip" android:layout_height="50dip" android:layout_below="@+id/center" android:layout_alignLeft="@+id/center" android:text="↓" /> <Button android:id="@+id/left" android:layout_width="50dip" android:layout_height="50dip" android:layout_toLeftOf="@+id/center" android:layout_alignTop="@+id/center" android:text="←" /> <Button android:id="@+id/right" android:layout_width="50dip" android:layout_height="50dip" android:layout_toRightOf="@+id/center" android:layout_alignTop="@+id/center" android:text="→" /> </RelativeLayout>
このレイアウトは、ビューをひとつだけ配置することを目的に設計された、もっともシンプルなレイアウトです。 配置されたビューは、通常一番左上を基点に配置されます。一番左上というのは、ビューの位置が(0, 0)に設定されることと同義です。 複数のビューを配置した場合、結果的に最後に配置したビューが最前面に描画された状態になります。
属性 | 意味 |
---|---|
forground | この属性に指定したイメージで最前面を多い被せるように描画する |
foregroudGravity | forgroundに指定したイメージの重力方向を指定する。指定できる値は、LinearLayoutと同様 |
mesureAllChildren | すべてのビューの寸法を計測する場合は、trueを指定する。デフォルトは、false |
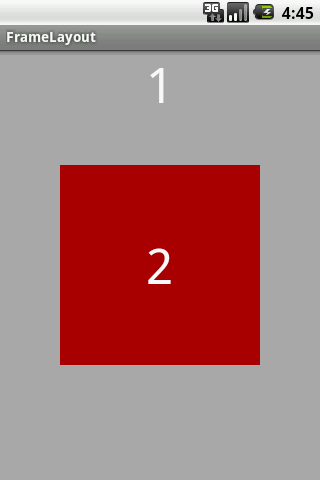
<?xml version="1.0" encoding="UTF-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/layout" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/white"> <TextView android:id="@+id/first" android:layout_width="fill_parent" android:layout_height="fill_parent" android:textColor="@android:color/white" android:background="@android:color/darker_gray" android:textSize="50sp" android:gravity="center_horizontal" android:text="1" /> <TextView android:id="@+id/sedond" android:layout_width="200dip" android:layout_height="200dip" android:background="#aa0000" android:layout_gravity="center" android:gravity="center" android:textSize="50sp" android:textColor="@android:color/white" android:text="2" /> </FrameLayout>
表形式で表現した場合に便利なレイアウトです。 1行を表すTableRowオブジェクトのリストで構成されます。 表の列数は、そのTableRowオブジェクトのなかで一番多いセル数がカラム数として採用されます。
属性 | 意味 |
---|---|
collapseColumns | 指定したインデックスに対応するカラムを非表示にする |
shrinkColumns | 指定したインデックスに対するカラムを可能な限り縮める |
stretchColumns | 指定したインデックスに対するカラムを可能な限り伸ばす |
layout_column | テーブルの列数よりも少ないセルを設定した場合、そのセルを何番目から表示するのか指定する |
layout_span | セルを結合する。HTMLタグのcolspan属性と同義 |
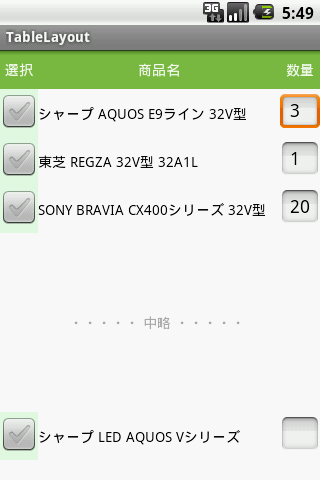
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@android:color/white" android:shrinkColumns="2" android:stretchColumns="0,1"> <TableRow android:layout_width="fill_parent" android:paddingTop="10dip" android:paddingBottom="10dip" android:background="#78bc40"> <TextView android:text="選択" android:gravity="center" android:textColor="@android:color/white" /> <TextView android:text="商品名" android:gravity="center" android:textColor="@android:color/white" /> <TextView android:text="数量" android:gravity="center" android:textColor="@android:color/white" /> </TableRow> <TableRow> <CheckBox android:background="#e0ffe0" /> <TextView android:textColor="@android:color/black" android:text="シャープ AQUOS E9ライン 32V型 " /> <EditText android:layout_width="40dip" android:layout_height="40dip" android:numeric="integer" android:singleLine="true" android:text="3"/> </TableRow> <TableRow> <CheckBox android:background="#e0ffe0" /> <TextView android:textColor="@android:color/black" android:text="東芝 REGZA 32V型 32A1L" /> <EditText android:layout_width="40dip" android:layout_height="40dip" android:numeric="integer" android:singleLine="true" android:text="1"/> </TableRow> <TableRow> <CheckBox android:background="#e0ffe0" /> <TextView android:textColor="@android:color/black" android:text="SONY BRAVIA CX400シリーズ 32V型 " /> <EditText android:layout_width="40dip" android:layout_height="40dip" android:numeric="integer" android:singleLine="true" android:text="20" /> </TableRow> <TableRow> <TextView android:layout_column="1" android:gravity="center" android:layout_marginTop="80dip" android:layout_marginBottom="80dip" android:textColor="@android:color/darker_gray" android:text="・・・・・ 中略 ・・・・・ " /> </TableRow> <TableRow> <CheckBox android:background="#e0ffe0" /> <TextView android:textColor="@android:color/black" android:text="シャープ LED AQUOS Vシリーズ" /> <EditText android:layout_width="40dip" android:layout_height="40dip" android:numeric="integer" android:singleLine="true" android:text="" /> </TableRow> </TableLayout>
このページへのコメント
cKPzXg <a href="http://thlcjmyaffuk.com/">thlcjmyaffuk</a>, [url=http://yxiphkpxjizw.com/]yxiphkpxjizw[/url], [link=http://wvyfrwejwdjo.com/]wvyfrwejwdjo[/link], http://pwcxpjmzanwr.com/
calculi mader opportunity
http://download.cyberlink.powerdvd.deluxe.8.0.soft...
zQJ704 <a href="http://zjspxxpneabi.com/">zjspxxpneabi</a>, [url=http://korfnmplwqad.com/]korfnmplwqad[/url], [link=http://acolifeykdlg.com/]acolifeykdlg[/link], http://nsamzcegbgfg.com/
http://fjvcjamxbjnr.com/
gq3zAw <a href="http://acuxqwobriji.com/">acuxqwobriji</a>, [url=http://yojtyjyqczjn.com/]yojtyjyqczjn[/url], [link=http://hkkrwtszinpy.com/]hkkrwtszinpy[/link], http://tvjajjqqrkqe.com/
IiI1ap <a href="http://cbmwrxiunkea.com/">cbmwrxiunkea</a>, [url=http://cqnagvzetgzj.com/]cqnagvzetgzj[/url], [link=http://jsfatdlaqvlm.com/]jsfatdlaqvlm[/link], http://dufddrtjmajp.com/
http://okoxnbzaprej.com/