最終更新:
moonlight_aska 2011年10月16日(日) 22:46:40履歴
アラートダイアログは, 主に警告メッセージや確認メッセージを表示するために使用するダイアログである.
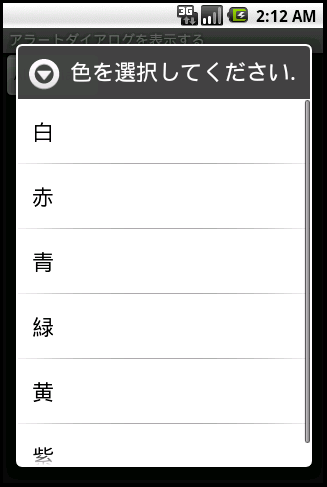
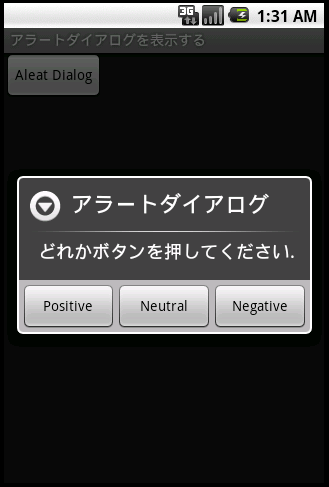
- AlertDialog01.java
- AlertDialog.Builderを生成する.
- setTitleメソッドでタイトルを設定する.
- setMessageメソッドで本文を設定する.
- setPositiveButton/setNeutralButton/setNegativeButtonメソッドでボタンに表示する文字列と, 押した時のリスナーを設定する.
- createメソッドでアラートダイアログを生成する.
- showメソッドでアラートダイアログを表示する.
package com.moonlight_aska.android.alertdialog01;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
public class AlertDialog01 extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button btn = (Button)findViewById(R.id.button_id);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
new AlertDialog.Builder(AlertDialog01.this)
.setTitle("アラートダイアログ")
.setMessage("どれかボタンを押してください.")
// 肯定的な意味を持つボタンを設定
.setPositiveButton("Positive", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
// Positive Buttonが押された時の処理を記述{
Log.v("Alert", "Positive Button");
}
})
// 中立的な意味を持つボタンを設定
.setNeutralButton("Neutral", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
// Neutral Buttonが押された時の処理を記述
Log.v("Alert", "Nuetral Button");
}
})
// 否定的な意味を持つボタンを設定
.setNegativeButton("Negative", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
// Negative Buttonが押された時の処理を記述
Log.v("Alert", "Negative Button");
}
})
.create()
.show();
}
});
}
}
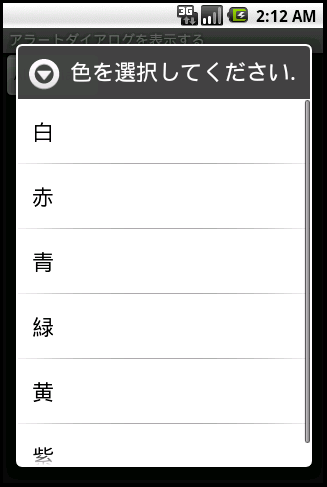
- AlertDialog02.java
- AlertDialog.Builderを生成する.
- setTitleメソッドでタイトルを設定する.
- setItemsメソッドでリスト表示する文字列の配列を設定する.
- createメソッドでアラートダイアログを生成する.
- showメソッドでアラートダイアログを表示する.
package com.moonlight_aska.android.alertdialog02;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
public class AlertDialog02 extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button btn = (Button)findViewById(R.id.button_id);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
// リスト表示する文字列
final String[] ITEM = new String[]{"白", "赤", "青", "緑", "黄", "紫"};
new AlertDialog.Builder(AlertDialog02.this)
.setTitle("色を選択してください.")
.setItems(ITEM, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
// アイテムが選択されたときの処理. whichが選択されたアイテムの番号.
Log.v("Alert", "Item No : " + which);
}
})
.create()
.show();
}
});
}
}
コメントをかく