最終更新:
moonlight_aska 2014年09月28日(日) 17:17:56履歴
比較的重い処理(画像処理, DBアクセス, HTTPダウンロード等)をUIスレッドに負荷を与えずバックグラウンドで処理したい場合, IntentServiceクラスを利用する.
他にも「AsyncTaskで非同期処理を行う」でも実現できる.
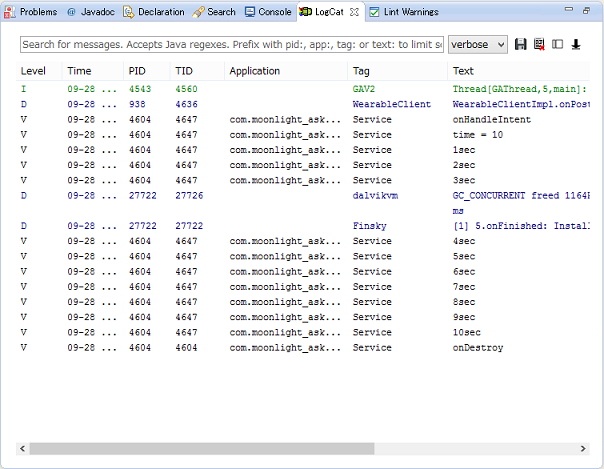
他にも「AsyncTaskで非同期処理を行う」でも実現できる.
- src/SampleIntentService.java
- IntentService#onHandleIntentメソッド内に非同期処理を実装する.
- Intent#getXXXExtraメソッドでActivityからのデータを受け取る.
package com.moonlight_aska.android.intentservice01;
import android.app.IntentService;
import android.content.Intent;
import android.util.Log;
public class SampleIntentService extends IntentService {
static String TAG = "Service";
public SampleIntentService() {
super("IntentService");
// TODO Auto-generated constructor stub
}
// バックグラウンド処理
@Override
protected void onHandleIntent(Intent intent) {
// TODO Auto-generated method stub
try {
Log.v(TAG, "onHandleIntent");
// データを受け取る
int time = intent.getIntExtra("SetTime", 0);
Log.v(TAG, "Time = " + time);
for (int i=0; i<time; i++) {
Thread.sleep(1000);
Log.v(TAG, (i+1) + "sec");
}
} catch (InterruptedException e){
e.printStackTrace();
}
}
@Override
public void onDestroy() {
// TODO Auto-generated method stub
Log.v(TAG, "onDestroy");
super.onDestroy();
}
}
- src/MainActivity.java
- SampleIntentService.classを指定して, インテントのインスタンスを生成する.
- Intent.putExtraメソッドで, データをインテントに渡す
- Context.startServiceメソッドで, インテントを起動する.
package com.moonlight_aska.android.intentservice01;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity {
private Context mContext = this;
private Button mBtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mBtn = (Button)findViewById(R.id.btn_id);
// Buttonイベント
mBtn.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
// Intentのインスタンスを生成
Intent intent = new Intent(Intent.ACTION_SYNC, null, mContext, SampleIntentService.class);
// データを渡す
intent.putExtra("SetTime", 10);
// インテントを起動
startService(intent);
}
});
}
}
- AndroidManifest.xml
- SampleIntentServiceを登録する.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.moonlight_aska.android.intentservice01"
andoid:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="21" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name="SampleIntentService"></service>
</application>
</manifest>
- 動作例
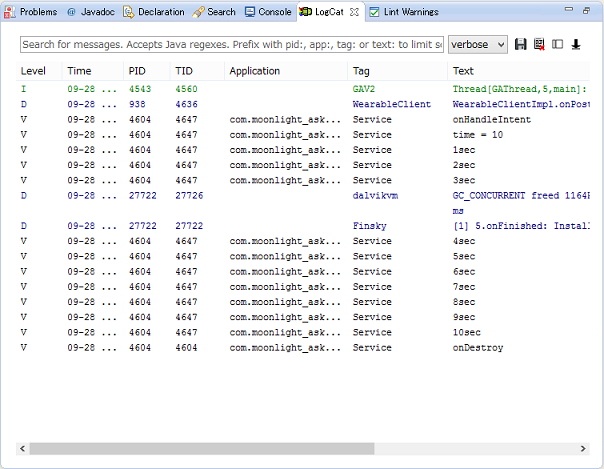
コメントをかく